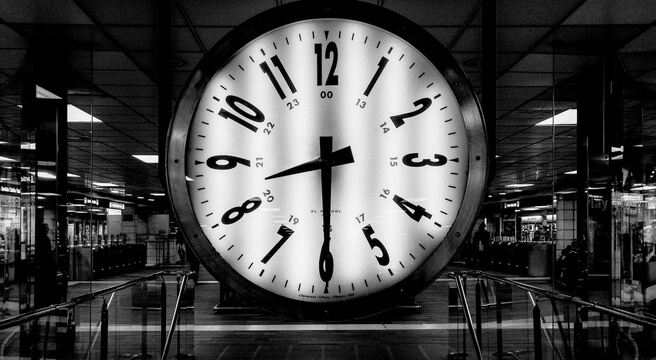
You can use Python’s datetime module to get the current date and time and then format it in the desired format (YYYYMMDDHHMMSS). Here’s an example:
from datetime import datetime
# Get the current date and time
current_datetime = datetime.now()
# Format the date and time in YYYYMMDDHHMMSS format
formatted_datetime = current_datetime.strftime('%Y%m%d%H%M%S')
print(formatted_datetime)
Running this code will print the current date and time in the form “YYYYMMDDHHMMSS”
In Python, there are several ways to work with timestamps, representing time points. Here are some commonly used timestamp representations and modules for working with them:
Unix Timestamp (Epoch Time)
Unix timestamp is the number of seconds that have passed since January 1, 1970, at 00:00:00 UTC. It is a widely used timestamp format for many programming languages.
time.time() # Returns the current Unix timestamp as a floating-point number.
datetime.datetime.timestamp() # Converts a datetime object to a Unix timestamp.
datetime Module
The built-in datetime module provides classes for representing dates and times. It offers various methods for working with timestamps.
datetime.datetime.now() # Returns a datetime object representing the current date and time.
datetime.datetime.utcnow() # Returns a datetime object representing the current UTC date and time.
datetime.datetime.fromtimestamp() # Converts a Unix timestamp to a datetime object.
Time Module
The time module provides functions for working with time-related operations.
time.time() # Returns the current time in seconds since the epoch (Unix timestamp).
time.localtime() # Returns a named tuple representing the local time.
time.gmtime() # Returns a named tuple representing the UTC time.
calendar Module
The calendar module provides functions related to calendar operations and time conversions.
calendar.timegm() # Converts a struct_time tuple (UTC) to a Unix timestamp.
Remember that when working with timestamps, you’ll need to consider factors like time zones, daylight saving time, and formatting. Choose the appropriate method or module based on your needs and the complexity of your timestamp-related tasks.
The datetime module
The datetime module is a part of Python’s standard library and it provides several classes such as datetime, date, time, timedelta, and timezone, that are used for handling dates and times. The datetime class is used to represent a particular point in time, while the date and time classes are used to represent only the date or time components, respectively. The timedelta class calculates time differences, and the timezone class handles time zone information. This module is commonly used for tasks that involve date and time manipulation, and it offers various functionalities like creating, formatting, and performing arithmetic operations on dates and times.