PowerShell is a powerful scripting language and command-line shell developed by Microsoft. It comes with a rich set of commands, known as cmdlets, which allow you to perform various tasks in the Windows operating system and other Microsoft products.
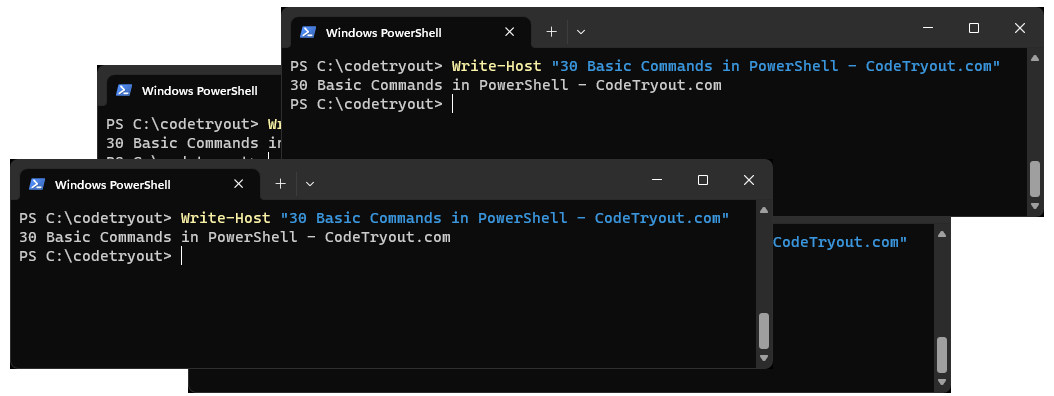
Here are 30 basic Commands(cmdlets) examples in PowerShell:
#1 Clear-Host: Clears the current PowerShell console window.
Here is an example of using the Clear-Host cmdlet in PowerShell
# codetryout.com PowerShell command demo script
Clear-Host
#2 Copy-Item: Copies a file or folder to a specified destination.
Here is an example of using the Copy-Item cmdlet in PowerShell
# codetryout.com PowerShell command demo script
# Source file path
$sourcePath = "C:\Path\to\source\codetryout.txt"
# Destination file path
$destinationPath = "C:\Path\to\destination\codetryout.txt"
# Copy the file
Copy-Item -Path $sourcePath -Destination $destinationPath
# Display a message once the file is copied
Write-Host "File copied successfully!"
#3 Export-ModuleMember: Specifies which module members are exported.
Here is an example of using the Export-ModuleMember cmdlet in PowerShell
# codetryout.com PowerShell command demo script
# Variable
$MyVariable = "Hello Codetryout!"
# Export the variable
Export-ModuleMember -Variable MyVariable
# To Export a user defined function, you can use
Export-ModuleMember -Function YourFunctionName
#4 ForEach-Object: Operates on each item in a collection.
Here is an example of using the ForEach-Object cmdlet in PowerShell
# codetryout.com PowerShell command demo script
# Create an array of numbers
$numbers = 1..5
# Use ForEach-Object to process each number
$numbers | ForEach-Object {
$result = $_ * 2
Write-Host "Result: $result"
}
When you run this code, you will see the following output:
Result: 2
Result: 4
Result: 6
Result: 8
Result: 10
#5 Get-ChildItem: Lists files and folders in the current directory.
Here is an example of using the Get-ChildItem cmdlet in PowerThe Get-ChildItem cmdlet in PowerShell is used to retrieve a list of child items (files and directories) within a specified location. Here’s an example:
# codetryout.com PowerShell command demo script
# Retrieve a list of files and directories in the current directory
$items = Get-ChildItem
# Loop through each item and display its name and type
foreach ($item in $items) {
Write-Host "Name: $($item.Name), Type: $($item.GetType().Name)"
}
#6 Get-Content: It reads the content of a file and displays it in the console.
The Get-Content cmdlet in PowerShell is used to read the contents of a file and return them as a collection of strings. Here’s an example:
# codetryout.com PowerShell command demo script
# Read the contents of a text file
$filePath = "C:\Path\to\file.txt"
$content = Get-Content -Path $filePath
# Loop through each line and display it
foreach ($line in $content) {
Write-Host $line
}
#7 Get-Help: Provides help information for PowerShell commands.
The Get-Help cmdlet in PowerShell retrieves detailed information about a cmdlet, function, script, or concept. Here are some examples:
# codetryout.com PowerShell command demo script
# Get help for the Get-Process cmdlet
Get-Help Get-Process
# Get help for the -Name parameter of Get-Process cmdlet
Get-Help Get-Process -Parameter Name
# Get examples for using Get-Process cmdlet
Get-Help Get-Process -Examples
#8 Get-Item: Retrieves information about a file or folder.
Here is an example of using the Get-Item cmdlet in PowerShell
# codetryout.com PowerShell command demo script
# Retrieve information about a directory to $directoryItem
$directoryItem = Get-Item -Path "C:\Path\to\directory"
# To display information about the directory
Write-Host "Name: $($directoryItem.Name)"
Write-Host "Parent Path: $($directoryItem.Parent.FullName)"
Write-Host "Creation Time: $($directoryItem.CreationTime)"
Write-Host "Last Access Time: $($directoryItem.LastAccessTime)"
Write-Host "Attributes: $($directoryItem.Attributes)"
You can modify the example by replacing the directory path with the path to your desired directory. Additionally, you can use Get-Item to retrieve information about files, registry keys, certificates, and other items depending on the provider supported by the PowerShell environment.
#9 Get-Module: Retrieves information about modules that are currently loaded.
Here is an example of using the Get-Module cmdlet in PowerShell
# codetryout.com PowerShell command demo script
# Retrieve information about all loaded modules
$modules = Get-Module
# Display information about each loaded module
foreach ($module in $modules) {
Write-Host "Module Name: $($module.Name)"
Write-Host "Version: $($module.Version)"
Write-Host "Path: $($module.Path)"
Write-Host "Exported Functions: $($module.ExportedFunctions.Count)"
Write-Host "Exported Cmdlets: $($module.ExportedCmdlets.Count)"
Write-Host "Exported Variables: $($module.ExportedVariables.Count)"
Write-Host
}
#10 Get-Process: Retrieves information about running processes on the system.
Here is an example of using the Get-Process cmdlet in PowerShell
# codetryout.com PowerShell command demo script
# Retrieve information about all running processes
Get-Process
# Retrieve information about a specific process
Get-Process -Name "chrome"
# Retrieve information about processes running on a remote computer
Get-Process -ComputerName "RemoteComputerName"
# Retrieve information about processes and sort them by CPU usage
Get-Process | Sort-Object -Property CPU
# Retrieve information about processes and filter them based on a condition
Get-Process | Where-Object { $_.CPU -gt 50 }
Get-Help Get-Process -Full will display the complete help documentation for the Get-Process cmdlet, including detailed information about its parameters, examples, and usage.
#11 Get-Service: Displays the status of services running on the system.
Here is an example of using the Get-Service cmdlet in PowerShell
# codetryout.com PowerShell command demo script
# Retrieve all services on the local computer
$services = Get-Service
# Display the name and status of each service
foreach ($service in $services) {
Write-Host "Service Name: $($service.Name), Status: $($service.Status)"
}
#12 Get-Variable: Retrieves information about variables in the current session.
Here is an example of using the Get-Variable cmdlet in PowerShell
# codetryout.com PowerShell command demo script
# Define some variables
$myString = "Hello, World!"
$myNumber = 42
$myArray = @(1, 2, 3)
# Retrieve information about all variables
$variables = Get-Variable
# Display information about each variable
foreach ($variable in $variables) {
Write-Host "Variable Name: $($variable.Name)"
Write-Host "Value: $($variable.Value)"
Write-Host "Type: $($variable.Value.GetType().Name)"
Write-Host
}
#13 Import-Module: Imports a module into the current session.
Here is an example of using the Import-Module cmdlet in PowerShell
# codetryout.com PowerShell command demo script
# Import a module named "MyModule"
Import-Module -Name MyModule
#14 Invoke-Command: Runs commands on remote computers.
Here is an example of using the Invoke-Command cmdlet in PowerShell
# codetryout.com PowerShell command demo script
# Run a simple command on a remote computer
Invoke-Command -ComputerName "RemoteComputerName" -ScriptBlock { Get-Process }
# Run a script file on a remote computer
Invoke-Command -ComputerName "RemoteComputerName" -FilePath "C:\codetryout\devops.ps1"
#15 Export-Csv.
The Export-Csv cmdlet in PowerShell is used to export data to a CSV (Comma-Separated Values) file format. Here’s an example:
# codetryout.com PowerShell command demo script
# Create an array of objects representing data
$data = @(
[PSCustomObject]@{
Name = "John Doe"
Age = 30
City = "New York"
},
[PSCustomObject]@{
Name = "Jane Smith"
Age = 25
City = "London"
}
)
# Export the data to a CSV file
$data | Export-Csv -Path "C:\Path\to\output.csv"
The above script would create a file C:\codetryout\output.csv with the following content:
#TYPE System.Management.Automation.PSCustomObject
"Name","Age","City"
"Dev Ops","30","New York"
"Code Tryout","25","London"
If you would like to skip printing the header line to the output file, you can change the last line as
$data | Export-Csv -Path "C:\Path\to\output.csv" -NoTypeInformation
Related: Parsing CSV in Python
#16 Move-Item: Moves a file or folder to a specified destination.
Here is an example of using the Move-Item cmdlet in PowerShell
# codetryout.com PowerShell command demo script
# Move a file to a new location
Move-Item -Path "C:\codetryout\Source\File.txt" -Destination "D:\codetryout\Destination\"
# Move a folder to a new location
Move-Item -Path "C:\codetryout\Source\FolderName" -Destination "D:\codetryout\Destination\"
#17 New-Alias: Creates a new alias for a command or cmdlet.
Here is an example of using the New-Alias cmdlet in PowerShell
# codetryout.com PowerShell command demo script
# Create an alias for the Get-Process cmdlet
New-Alias -Name "gp" -Value "Get-Process"
# Use the alias to run the Get-Process cmdlet
gp
# Create an alias for a script file
New-Alias -Name "startscript" -Value "C:\codetryout\devops.ps1"
# Use the alias to run the script file
startscript
# Create a temporary alias for a command
New-Alias -Name "ls" -Value "Get-ChildItem" -Option "ReadOnly"
# Try to overwrite the existing ls alias with a new value
New-Alias -Name "ls" -Value "Set-Location" -Force
# Remove an alias
Remove-Item -Path "Alias:ls"
#18 New-Item: Creates a new file or folder.
Here is an example of using the New-ItemNew-Items cmdlet in PowerShell
# codetryout.com PowerShell command demo script
# Create a new text file
New-Item -Path "C:\Path\to\file.txt" -ItemType "File"
# Create a new directory
New-Item -Path "C:\Path\to\newfolder" -ItemType "Directory"
#19 New-Module: Creates a new module.
Here is an example of using the New-Module cmdlet in PowerShell
# codetryout.com PowerShell command demo script
# Create a new module with a manifest file
New-Module -Name "MyModule" -ScriptBlock {
function Get-Greeting {
param (
[string]$Name
)
Write-Output "Hello, $Name!"
}
} -ModuleVersion "1.0" -OutputModulePath "C:\codetryout\modules"
To import the newly created module
# Import the newly created module
Import-Module -Name "C:\codetryout\modules\MyModule" -Verbose
# Use the function from the module
Get-Greeting -Name "DevOps"
#20 Remove-Item: Deletes a file or folder.
Here is an example of using the Remove-Item cmdlet in PowerShell
# codetryout.com PowerShell command demo script
# To remove a file
Remove-Item -Path <PathOrName>
# To remove a folder
Remove-Item -Path "C:\codetryout\devops_test"
The above line of command will ask for confirmation if the folder is not empty. To delete without asking for validation:
Remove-Item -Path "C:\codetryout\devops_test" -Confirm:$false
#21 Rename-Item: Renames a file or folder.
Here is an example of using the Rename-Item cmdlet in PowerShell
# codetryout.com PowerShell command demo script
#22 Set-Content: Writes content to a file.
Here is an example of using the Set-Content cmdlet in PowerShell
# codetryout.com PowerShell command demo script
Rename-Item -Path <CurrentPathOrName> -NewName <NewName>
#23 Set-Location: Changes the current directory.
Here is an example of using the Set-Location cmdlet in PowerShell
# codetryout.com PowerShell command demo script
Set-Location -Path <Path>
#24 Sort-Object: Sorts objects based on specified properties.
Here is an example of using the Sort-Object cmdlet in PowerShell
# codetryout.com PowerShell command demo script
$numbers = 4, 2, 6, 1, 3
$sortedNumbers = $numbers | Sort-Object
$sortedNumbers
#25 Start-Process: Starts a new process.
Here is an example of using the Start-Process cmdlet in PowerShell
# codetryout.com PowerShell command demo script
Start-Process -FilePath "C:\Path\to\executable.exe" -ArgumentList "-arg1 value1 -arg2 value2" -WorkingDirectory "C:\Path\to\working\directory"
#26 Stop-Process: Stops one or more processes.
Here is an example of using the Stop-Process cmdlet in PowerShell.
# codetryout.com PowerShell command demo script
Stop-Process [-ID] <ProcessID> [-Force] [-PassThru]
Stop-Process [-Name] <ProcessName> [-Force] [-PassThru]
Stopping a process by process ID
# codetryout.com PowerShell command demo script
Stop-Process -ID 1234 -Force
#27 Test-Path: Checks if a file or folder exists at a specified location.
Here is an example of using the Test-Path cmdlet in PowerShell
# codetryout.com PowerShell command demo script
$path = "C:\codetryout\file.txt"
if (Test-Path $path) {
Write-Host "The file exists."
} else {
Write-Host "The file does not exist."
}
#28 Where-Object: Filters objects based on specified criteria.
The Where-Object cmdlet in PowerShell filters objects based on specified conditions. It allows you to select objects that meet specific criteria. Here’s the basic syntax:
# codetryout.com PowerShell command demo script
<Objects> | Where-Object <FilterExpression>
Example of filtering running Chrome process
# codetryout.com PowerShell command demo script
$processes = Get-Process
$filteredProcesses = $processes | Where-Object { $_.Name -eq "chrome" }
$filteredProcesses
#29 Write-Host: Displays output in the console.
Here is an example of using the Write-Host cmdlet in PowerShell
# codetryout.com PowerShell command demo script
Write-Host "Hello, CodeTryout World!"
#30 Write-Output: Sends output to the pipeline.
Here is an example of using the Write-Output cmdlet in PowerShell
# codetryout.com PowerShell command demo script
Write-Output "Hello, World!"
You can also capture the output of Write-Output in a variable for further processing. Here’s an example:
# codetryout.com PowerShell command demo script
$output = Write-Output "This is a test"
These are just a few examples of basic PowerShell commands. PowerShell is a versatile scripting language, and numerous other cmdlets and functionalities are available for various tasks. You can explore more commands and their usage using the Get-Help cmdlet or the official PowerShell documentation.