To parse a CSV file using Python, you can utilize the csv module, which provides functionality specifically for working with CSV files.
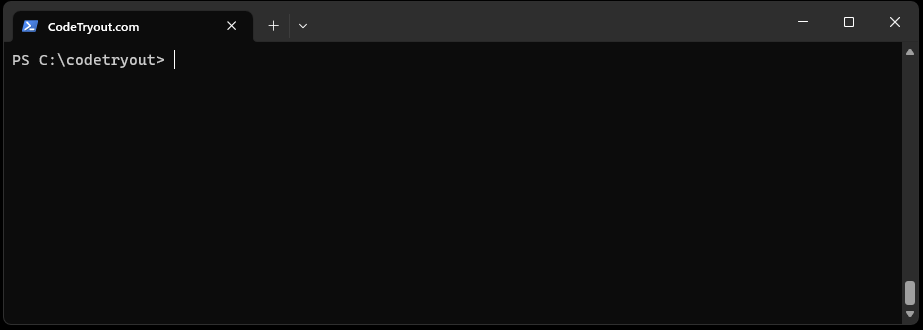
Here’s an example of how to parse a CSV (Comma-Separated Values) file using Python.
Example CSV
File: users.csv
"Name","Age","City"
"Dev Ops","30","New York"
"Code Tryout","25","London"
Python script, parsing the above CSV file
File: code.py
import csv
# Open the CSV file
with open('users.csv', 'r') as csv_file:
# Create a CSV reader object
csv_reader = csv.reader(csv_file)
# Iterate over each row in the CSV file
for row in csv_reader:
# Access data in each column of the row
column1 = row[0]
column2 = row[1]
# ... access other columns as needed
# Process or display the data
print(f'Column 1: {column1}, Column 2: {column2}')
Example Output:
PS C:\codetryout> python code.py
Column 1: Name, Column 2: Age
Column 1: Dev Ops, Column 2: 30
Column 1: Code Tryout, Column 2: 25
In this example, the csv module is imported, and the open() function is used to open the CSV file in read mode. The csv.reader function is then used to create a CSV reader object, which allows us to iterate over the rows in the CSV file.
Each row is accessed within the loop as a list, and the individual columns can be accessed using index numbers (starting from 0). You can assign the values to variables or perform any desired processing or analysis on the data.
The example code prints the values of each row’s first and second columns, but you can modify them to suit your needs.
Remember to replace ‘users.csv’ with your CSV file’s path and filename.
FAQs
What is a CSV File?
A CSV (Comma-Separated Values) file is a plain text file that stores tabular data in a structured format. It is commonly used for data storage and exchange between different software applications. In a CSV file, each line represents a row of data, and within each line, the values are separated by commas or other delimiters like semicolons or tabs.
CSV files can originate from various sources depending on the context and purpose of the data. Here are a few common ways CSV files are generated:
- Exporting data from spreadsheet software: CSV files are often created by exporting data from spreadsheet programs such as Microsoft Excel, Google Sheets, or LibreOffice Calc. Users can save their spreadsheet data in CSV format to make it easier to share, import into other applications, or work with programming languages.
- Database exports: Many database management systems provide the functionality to export data in CSV format. This allows users to extract data from databases and save it in an easily readable and transferable format. Database administrators or users with appropriate permissions can export tables or query results as CSV files.
- Web scraping: CSV files can be generated through web scraping, a technique used to extract data from websites. Web scraping tools or custom scripts can extract structured data from web pages and save it in CSV format for further analysis or integration with other systems.
- Data collection tools: Data collection tools, such as online surveys or form builders, often allow users to export collected data in CSV format. This enables users to download the collected data in a standardized format that can be easily processed and analyzed.
- Data transformations: CSV files can also be generated due to data transformations or conversions. For example, data may be extracted from one format (e.g., XML, JSON, or a database) and converted into CSV format to simplify the data structure or make it more suitable for specific applications or analyses.
Why parse CSV in Python?
Parsing CSV files in Python is necessary to read and extract data from CSV files and work with it programmatically.