Welcome to the world of Python programming! Whether you are taking your first steps in the programming journey or looking for practical examples to reinforce your understanding, these simple Python programs are designed with beginners in mind. Each program covers fundamental concepts and allows one to practice basic coding skills.
As you explore these examples, you’ll encounter programs ranging from a basic calculator to a simple to-do list. These programs touch upon various aspects of Python, including user input, conditionals, loops, mathematical operations, and even list manipulation. Feel free to run and modify the code, experiment with different inputs, and observe how the programs respond.
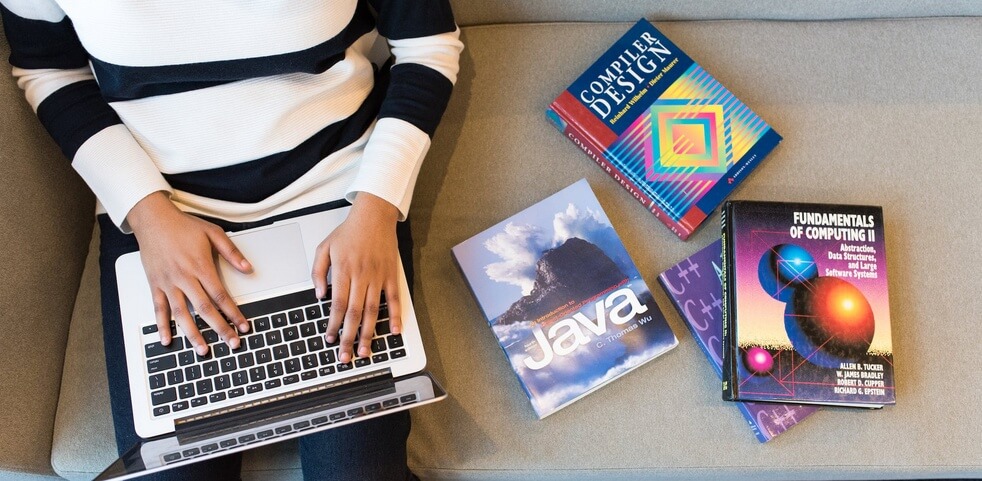
Remember, programming is about writing code and understanding how to think logically and solve problems. Take your time to explore and enjoy the process of learning Python programming with these hands-on examples. Happy coding!
Here are seven simple Python programs (scripts) for beginners:
Simple calculator program
# Simple calculator program
# Get input from the user
num1 = float(input("Enter the first number: "))
operator = input("Enter the operator (+, -, *, /): ")
num2 = float(input("Enter the second number: "))
# Perform calculation based on the operator
if operator == "+":
result = num1 + num2
elif operator == "-":
result = num1 - num2
elif operator == "*":
result = num1 * num2
elif operator == "/":
result = num1 / num2
else:
result = "Invalid operator"
# Print the result
print(f"Result: {result}")
Guess the number game
# Guess the number game
import random
# Generate a random number between 1 and 10
secret_number = random.randint(1, 10)
# Get input from the user
guess = int(input("Guess the number between 1 and 10: "))
# Check if the guess is correct
if guess == secret_number:
print("Congratulations! You guessed the correct number.")
else:
print(f"Sorry, the correct number was {secret_number}. Try again!")
Calculate the area of a circle
# Calculate the area of a circle
import math
# Get the radius from the user
radius = float(input("Enter the radius of the circle: "))
# Calculate the area of the circle
area = math.pi * radius ** 2
# Print the result
print(f"The area of the circle is: {area}")
Fahrenheit to Celsius converter
# Fahrenheit to Celsius converter
# Get the temperature in Fahrenheit from the user
fahrenheit = float(input("Enter the temperature in Fahrenheit: "))
# Convert Fahrenheit to Celsius
celsius = (fahrenheit - 32) * 5/9
# Print the result
print(f"The temperature in Celsius is: {celsius:.2f}°C")
List manipulation program
# List manipulation program
# Create a list of numbers
numbers = [1, 2, 3, 4, 5]
# Add a number to the list
numbers.append(6)
# Print the list
print("Original List:", numbers)
# Square each number in the list
squared_numbers = [num ** 2 for num in numbers]
# Print the squared numbers
print("Squared Numbers:", squared_numbers)
Factorial calculator
# Factorial calculator
# Get the number from the user
num = int(input("Enter a number to calculate its factorial: "))
# Calculate the factorial
factorial = 1
for i in range(1, num + 1):
factorial *= i
# Print the result
print(f"The factorial of {num} is: {factorial}")
Simple to-do list program
# Simple to-do list program
# Initialize an empty list for tasks
tasks = []
while True:
# Get user input for task or "exit" to quit
task = input("Enter a task or type 'exit' to quit: ")
if task.lower() == 'exit':
break # Exit the loop if the user types 'exit'
# Add the task to the list
tasks.append(task)
# Print the to-do list
print("\nYour To-Do List:")
for i, task in enumerate(tasks, 1):
print(f"{i}. {task}")