In Bash, the environment variable and a normal variable (a local variable within the script), are treated equally. If you want to check if the environment variable exists and is not empty, here is the way.
env check, one-liner for environment variable is set and not empty
The one-liner syntax to check would be:
[[ -z $myenv ]] && echo "NOT SET or empty" || echo "is SET and not empty"
Example one-liner below, where the variable is set by it is empty:

Now, let us set the variable and then retry the check again

env check script for checking if the environment variable exists and not empty
Now, let’s see how the same work in a full code snippet.
# bash check if environment variable is set and not empty
if [[ -z $pod_version ]]
then
echo "pod_version undefined!"
else
echo "The pod_version is: $pod_version"
fi
Running the script in the following scenarios
- Checking without defining the env variable
- Checking after defining the env variable
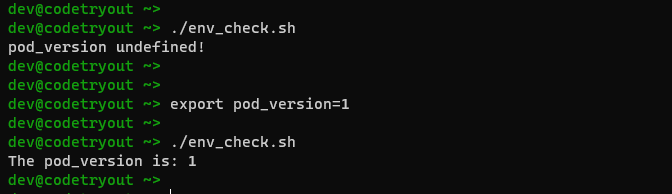
The export command used in this example is a bash built-in, that helps in making the environment variable available in the current and child shells and thus simulates an env-variable.
This is handy for your container ( example – docker or any other containers in pods ). You can use the full script or the oneliner depending upon your development strategies.