The requests module is a Python library used for making HTTP requests. It provides a simple way to interact with web services and retrieve data from APIs or websites. With the requests module, you can perform various HTTP operations such as sending GET, POST, PUT, DELETE, and other requests. It simplifies sending HTTP requests, handling headers, cookies, parameters, and response handling.
How to install requests in Python (Windows)
This guide will use Python Virtual environments to experiment with the requests module installations. You may skip the step for venv if you would like to. Otherwise, go to STEP #3
Step #1: Create a virtual environment (venv).
python -m venv codetryout_demo
Step #2: Switch to the new venv.
In this example, it is codetryout_demo
.\codetryout_demo\Scripts\activate
Step #3: Install requests Module
First – Testing whether the requests module is available in the venv
(codetryout_demo1) PS C:\> python -c "import requests"
Traceback (most recent call last):
File "<string>", line 1, in <module>
ModuleNotFoundError: No module named 'requests'
Installing requests module
pip install requests
Testing again after the module installation
(codetryout_demo) PS C:\> python -c "import requests"
(codetryout_demo) PS C:\>
How to install requests in Python on Windows – Demo:
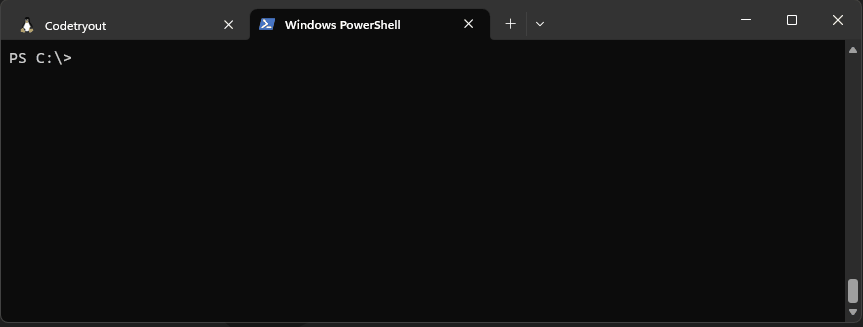
How to install requests in Python (Ubuntu)
This guide will use Python Virtual environments to experiment with the requests module installations. You may skip the step for venv if you would like to. Otherwise, go to STEP #3
Step #1: Create a virtual environment (venv)
python3 -m venv codetryout_venv1
This will create a virtual environment named codetryout_venv1.
Step #2: Activate the virtual environment: (Switching to the new virtual environment)
source codetryout_venv1/bin/activate
You will notice that the prompt in the terminal changes, indicating that you are now inside the virtual environment.
Step #3: Install the requests module
pip install requests
Testing the requests module:
dev@codetryout:~/py$ python3
Python 3.8.10 (default, May 26 2023, 14:05:08)
[GCC 9.4.0] on linux
Type "help", "copyright", "credits" or "license" for more information.
>>> import requests
>>>
How to deactivate the virtual environment?
When you’re done working, simply run:
deactivate
How to install requests in Python on Ubuntu – Demo:
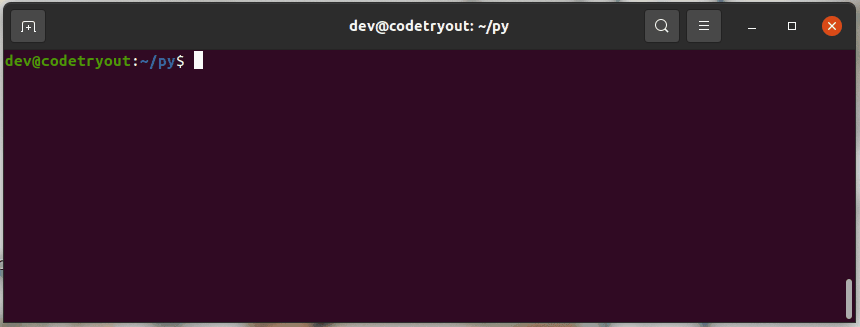
Requests module simple examples
You can use the request code to check whether the URL is responding. Response status code 200 means it is responding.
>>> import requests
>>> response = requests.get('https://codetryout.com/')
>>> print(response.status_code)
200
>>>
Here is a list of options available with response.
response.apparent_encoding
response.close
response.connection
response.content
response.cookies
response.elapsed
response.encoding
response.headers
response.history
response.is_permanent_redirect
response.is_redirect
response.iter_content
response.iter_lines
response.json
response.links
response.next
response.ok
response.raise_for_status
response.raw
response.reason
response.request
response.status_code
response.text
response.url
Related to creating a virtual environment Python